Working with devs and infrastructure to deliver your product – automating a basic task – Using Python for CI/CD Pipelines
Working with devs and infrastructure to deliver your product
Being a DevOps engineer means being the ultimate team player. It also means you have to socialize with and be somewhat liked by practically everyone on your team. And honestly, it’s not that hard; laugh at their awkward jokes and make a little small talk and suddenly you’re everyone’s friend. It’s not that hard to get people to work together if you want to. It is, however, quite difficult, some of the time, to get their efforts to coordinate with yours. This is why we have collaboration tools. Besides ordinary GitHub, we have all sorts of tools for whatever development model you are using. Jira, Slack, Zoom, Google Chat, Teams… I could go on forever. What happens quite often is that a lot of teams use multiple collaboration tools. So, the question then becomes, how do we get these collaboration tools to collaborate with each other?
There are a lot of connectors that the tools themselves provide, but sometimes their functionality needs to be facilitated with some code and making a few API calls. We will try this now using Python to connect two very common productivity tools: Todoist and Microsoft To Do. You may have heard about or used either one or both of these tools.
Todoist is a simple to-do list application. There’s nothing much to it; it is very similar to other such applications, such as Jira or Trello. Microsoft To Do is the same, except it is integrated into Microsoft 365.
Let’s start by extracting a list of tasks from the Todoist API. To do that, let’s create a Todoist account and add a few tasks from the UI:
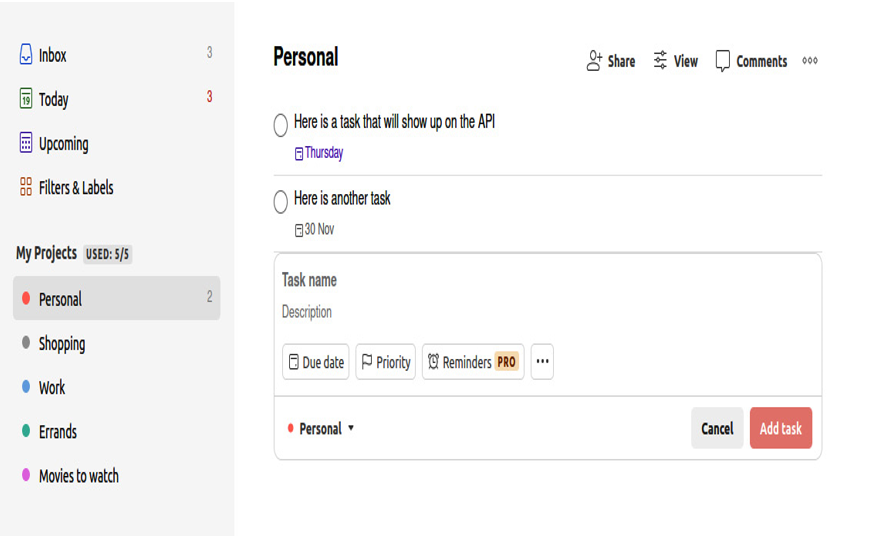
Figure 9.5 – Todoist dashboard
Just a couple of tasks with deadlines there. Now, let’s get the API token to call this API. Under your account’s Settings | Integrations | Developer tab, you will find the API key, which you can copy and use. Now, you can also install the Python todoist library:
pip install todoist_api_python
Next, write a script that integrates your API token and lists out all the tasks.
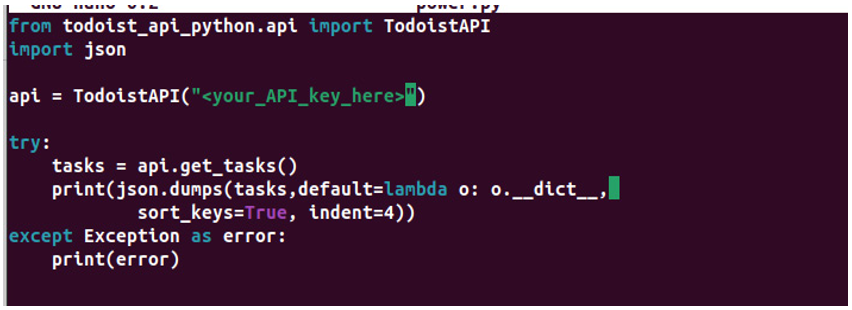
Figure 9.6 – Code to get Todoist tasks
Simple enough, with a little bit of syntactic sugar, it will get you a list of tasks like this:
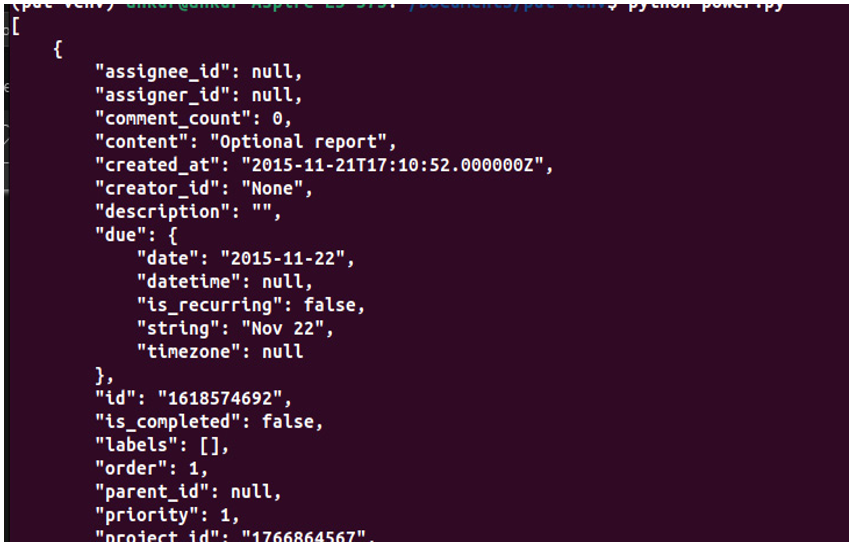
Figure 9.7 – Data extracted from the Todoist API
That’s just a small sample, but you can see the content, the description, and the due date, which are the things that we are interested in. Now, we are going to attempt to do the same thing with Microsoft To Do. For this, we are simply going to call the API endpoint. There is a library for Microsoft To Do in Python as well, but it is still in the experimental stage.
You will need to get an authorization token from Microsoft, which you can get by calling the API for it, as shown here: https://learn.microsoft.com/en-us/graph/auth-v2-user?context=graph%2Fapi%2F1.0&view=graph-rest-1.0&tabs=curl. I’m not including the process for this because it is quite clearly laid out here. You can integrate it into the code later if you want to automatically generate the token. Now, let’s modify our code in order to use the content, description, and due date that we have taken from Todoist:
from todoist_api_python.api import TodoistAPIimport jsonfrom datetime import datetime, timezoneimport requests#API tokensapi = TodoistAPI(“<your_api_token_here>”)access_token = “<Your_Microsoft_token_here>”#Endpoint for your default task listTODO_API_ENDPOINT = YOUR_TODO_ENDPOINThttps://graph.microsoft.com/v1.0/me/todo/lists/@default/tasks#Function to create task in Microsoft Tododef create_todo_task(title,date): headers = {‘Authorization’: ‘Bearer ‘+access_token, ‘Content-Type’: ‘application/json’} payload = {‘title’: title, ‘dueDateTime’: date} response = requests.post(TODO_API_ENDPOINT, headers=headers, json=payload) return response.json() if response.status_code == 201 else Nonetry: #Get task in JSON form tasks = json.dumps(api.get_tasks(),default=lambda o: o.__dict__,sort_keys=True, indent=4) #Parse tasks for task in tasks: title = task[“content”] date = task[“due”][“date”].replace(tzinfo=timezone.utc).astimezone(tz=None) #Create tasks based on information create_todo_task(title, date)except Exception as error: print(error)
And it’s as simple as that. We can use this code to take tasks from Todoist and put them in Microsoft To Do. We can even use the microservices-based architecture in our previous chapters (like in Chapter 8, Understanding Event-Driven Architecture) to make this even more efficient using webhooks and events. Speaking of events, in a lot of servers, one of the most common events is a failure. In the event of a failure, a rollback strategy is needed. Let’s see how Python can facilitate that.